In this page, you will learn to make your first Capillary API call.
Prerequisites:
- Authentication details - Till ID and password for basic auth and valid access token for OAuth.
- Appropriate access group access.
- API details
- Any API testing tool such as Postman, Swagger, etc.
Step-by-step instructions:
-
Determine the necessary API for your first request. Refer API Index for a list of our APIs.
-
Select an API tool. Below are some suggested tools along with guidance on their usage:
-
Build a URL for the API call. For more information, refer to the API URL structure documentation.
-
Select the type of API call. For information on API call types, refer to the API HTTP methods documentation.
-
Enter the authentication details. You can use Basic auth or OAuth. For more information refer to the Basic Auth and OAuth documentation.
Ensure that your till has the appropriate access group permissions. For example, if your till lacks write permissions for Customer resources, you will be unable to add a customer using those till credentials. For more information, refer to the access group. documentation.
-
Run the request.
-
Evaluate the response.
- For information on API response codes, refer API status codes documentation.
Once you have successfully made a request, make sure to check the response to ensure it is correct and as expected.
- For information on API response codes, refer API status codes documentation.
Getting started with Postman
Prerequisites:
- Authentication details - Till ID and password for basic auth and valid access token for OAuth.
- Appropriate access group access.
- API endpoint details.
Step by step instructions
- Open Postman and from the home page, click New .

- Select the Authorization tab and from the Type drop-down, select the authentication type. For basic authentication, select Basic auth and for authenticating using an access token, select No Auth.
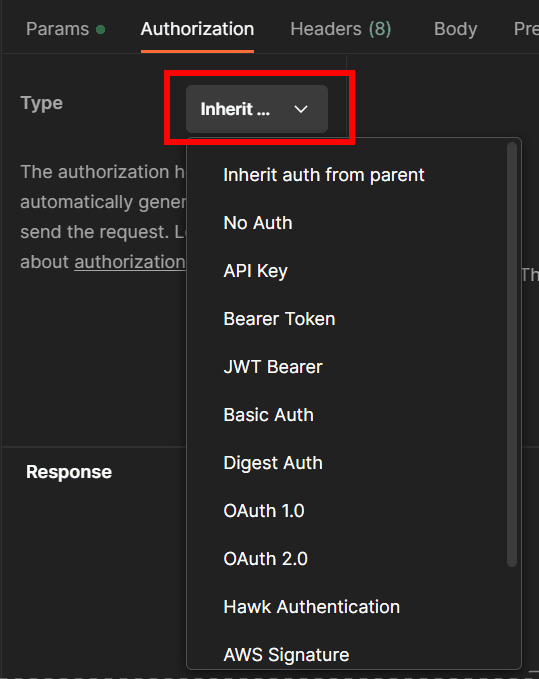
Basic Auth
To authenticate using basic auth, enter the Till ID in Username and in the Password field, enter the till password in MD5 format. For more information on these, refer Basic authentication documentation.
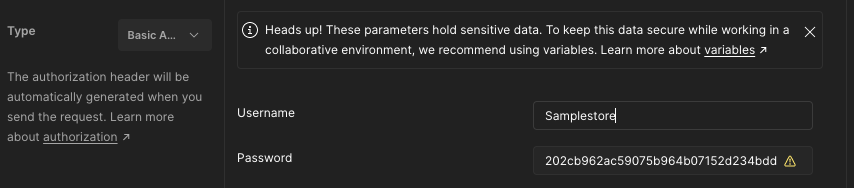
Authenticating using access token
To authenticate using an access token, generate the token, select Headers, and enter the Key X-CAP-API-OAUTH-TOKEN, then input the token in the Value field. The Content-Type and accept key fields are auto-generated.
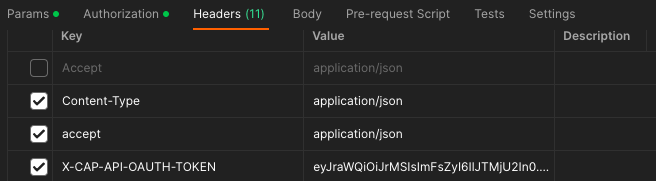
Warning
Ensure that your till has the appropriate access group permissions. For example, if your till lacks write permissions for Customer resources, you will be unable to add a customer using those till credentials. For more information, refer to the access group. documentation.
- From the method drop-down, select the method type.
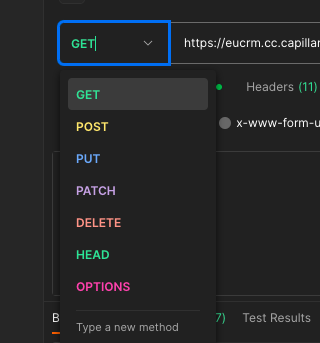
- In the URL text box, enter the API URL. Refer API Index for a list of our APIs and refer to the API URL structure documentation for information on building a URL.
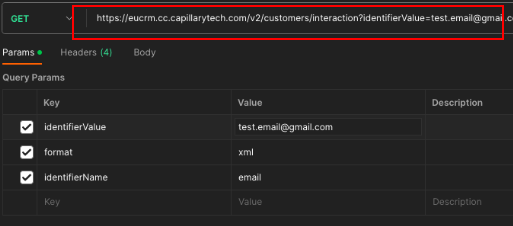
- (a) For POST and PUT methods, select Body tab > raw and enter the body parameters.
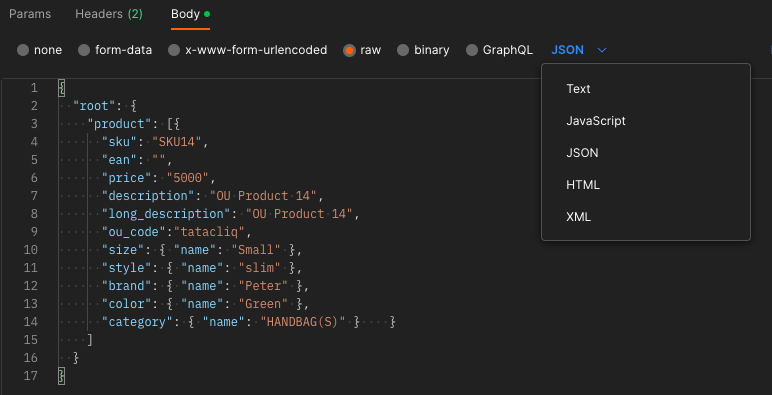
(b)For GET / DELETE methods, select the Parameters tab and enter the query parameters.
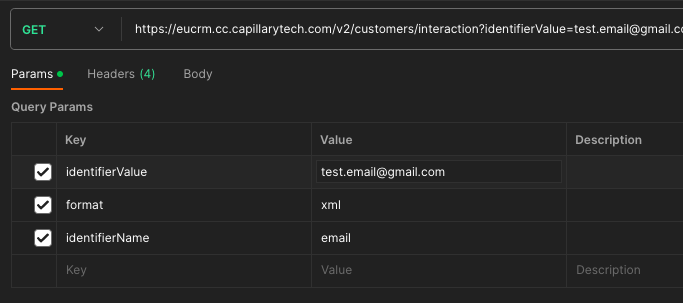
- Click Send. The response is displayed on the interface.
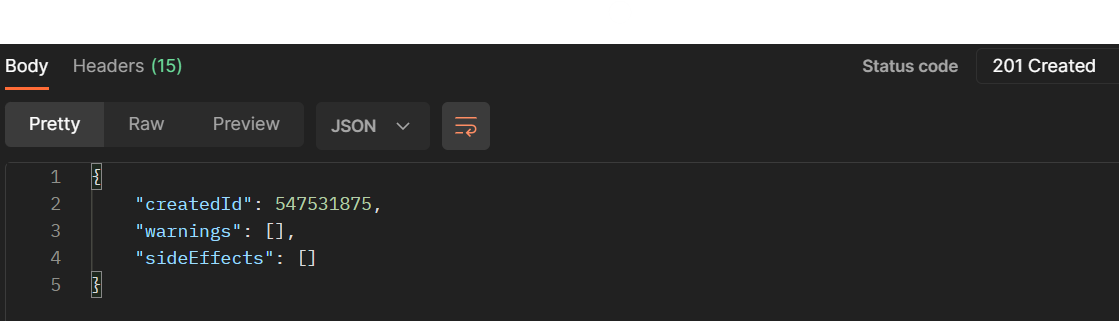
{
"loyaltyInfo": {
"loyaltyType": "loyalty"
},
"profiles": [
{
"firstName": "Jose",
"lastName": "Jones",
"identifiers": [
{
"type": "mobile",
"value": "988001234"
},
{
"type" : "email",
"value" : "[email protected]"
}
],
"source": "INSTORE"
}
]
}
//REQUEST
https://eucrm.cc.capillarytech.com/v2/customers/lookup?source=INSTORE&identifierName=mobile&identifierValue=9449489999
//RESPONSE
{
"entity": 552695692,
"warnings": []
}
// BODY
{
"profiles": [
{
"firstName": "Tom",
"lastName": "Sawyer",
"fields": {
"gender": "Male",
"city": "Bangalore"
},
"identifiers": [
{
"type": "mobile",
"value": 919111111111
},
{
"type": "email",
"value": "[email protected]"
},
{
"type": "wechat",
"value": "wc_2"
}
],
"commChannels": [
{
"type": "email",
"value": "[email protected]",
"primary": "true",
"verified": "false",
"meta": {
"residence": true
}
},
{
"type": "wechat",
"value": "wc_2",
"primary": "true",
"verified": "true",
"meta": {
"residence": true
}
},
{
"type": "mobilePush",
"value": "abcd12343434",
"primary": true,
"verified": true,
"meta": {
"lastViewedDate": "2019-10-25"
}
}
],
"source": "WECHAT",
"accountId": "1234"
}
],
"loyaltyInfo": {
"loyaltyType": "loyalty"
},
"extendedFields": {
"gender": "MALE",
"city": "Bangalore"
},
"loyaltyProgramEnrollments": [
{
"programId": 1016,
"tierNumber": 234,
"loyaltyPoints": 75,
"tierExpiryDate": "2022-02-11T16:36:17+05:30",
"pointsExpiryDate": "2022-02-11T16:36:17+05:30"
}
]
}
//RESPONSE
{
"createdId": 162116213,
"warnings": [],
"sideEffects": [
{
"awardedPoints": 25,
"type": "points"
}
]
}
//REQUEST
https://eucrm.cc.capillarytech.com/v2/userGroup2/1234/leave?source=INSTORE&accountId=321&identifierName=mobile&identifierValue=1234566789
//RESPONSE
{
"warnings": [],
"errors": [],
"success": true
}
You can click Save as example to save the response for future reference.
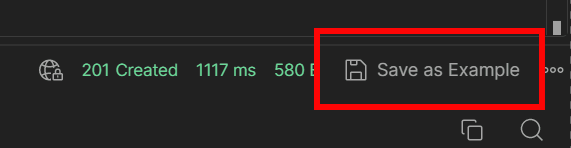
Using Postman for Bulk API requests
You can configure automation for repetitive API requests in Postman. This approach allows the automatic execution of numerous API requests and is useful when there is no specific BULK API available for your requirements.
Note
This method is primarily intended for cleanup activities during migrations or other rare circumstances. It is not recommended for all APIs and should not be considered a replacement for BULK APIs.
To create a Bulk API request using POSTMAN, perform the following:
- Click on the Add icon.
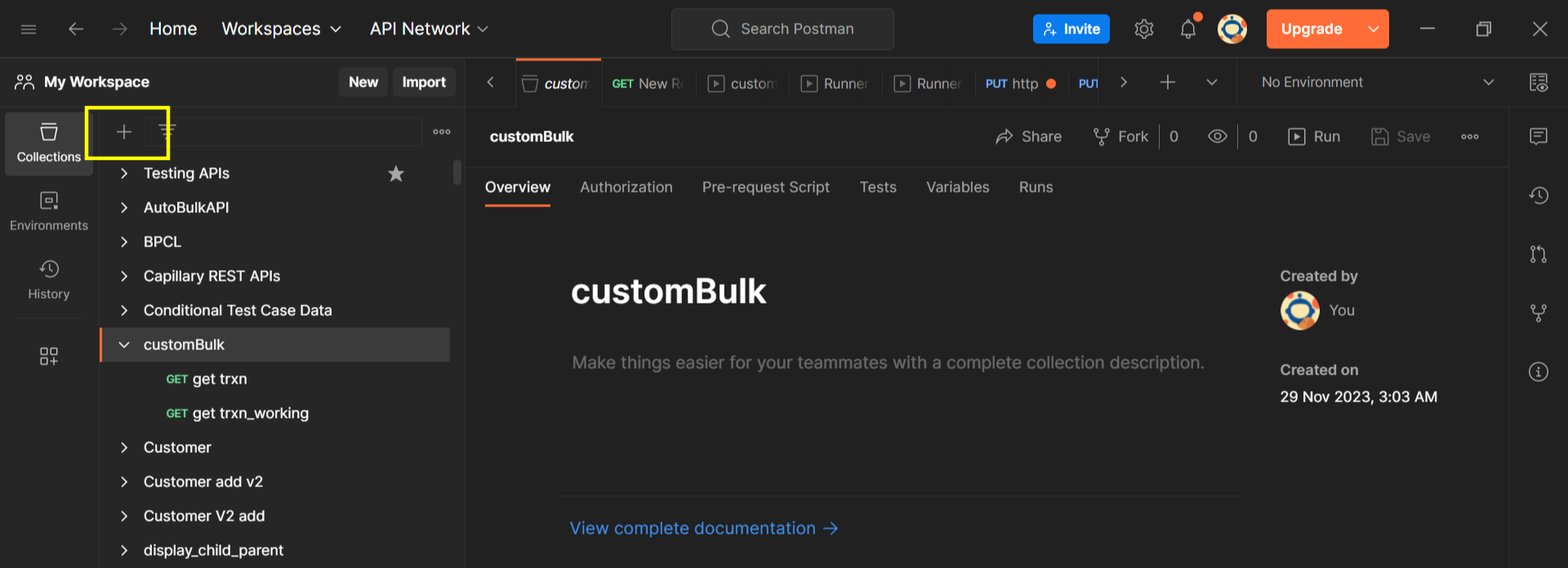
- Click Blank collection. A new collection is created.
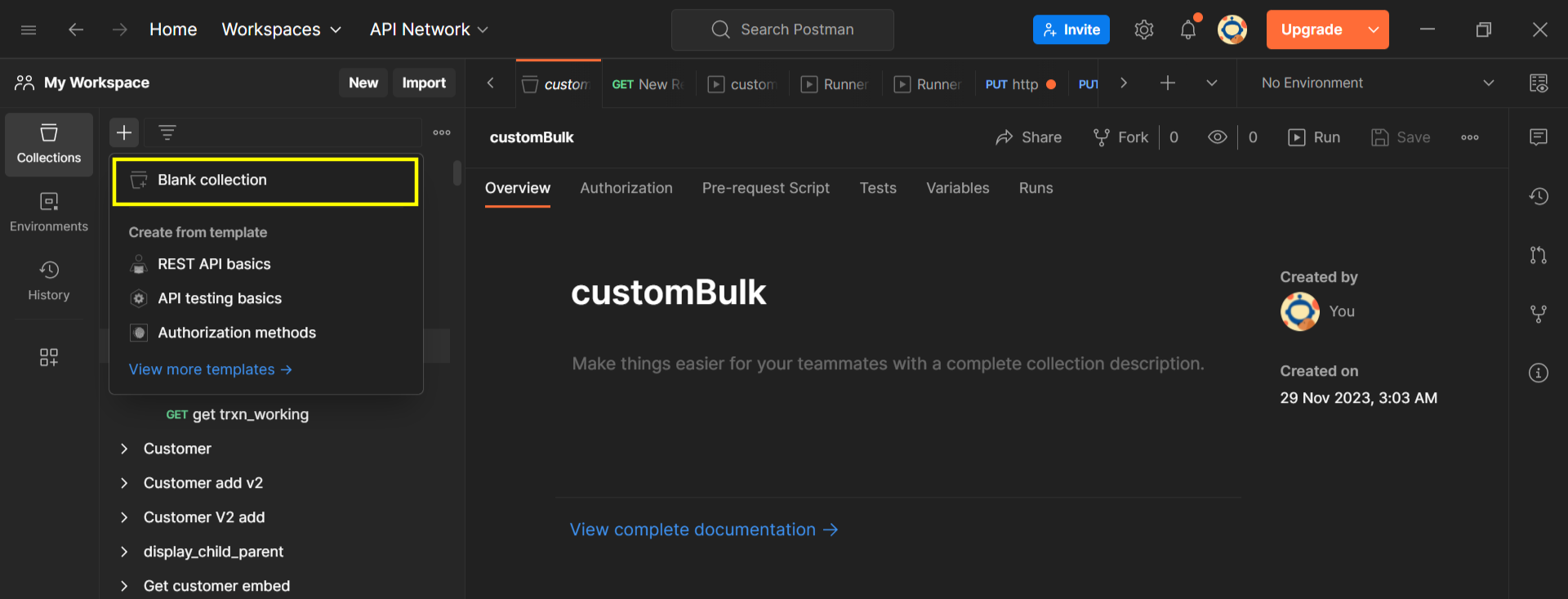
- Click the three-dot menu, and click Add a request.
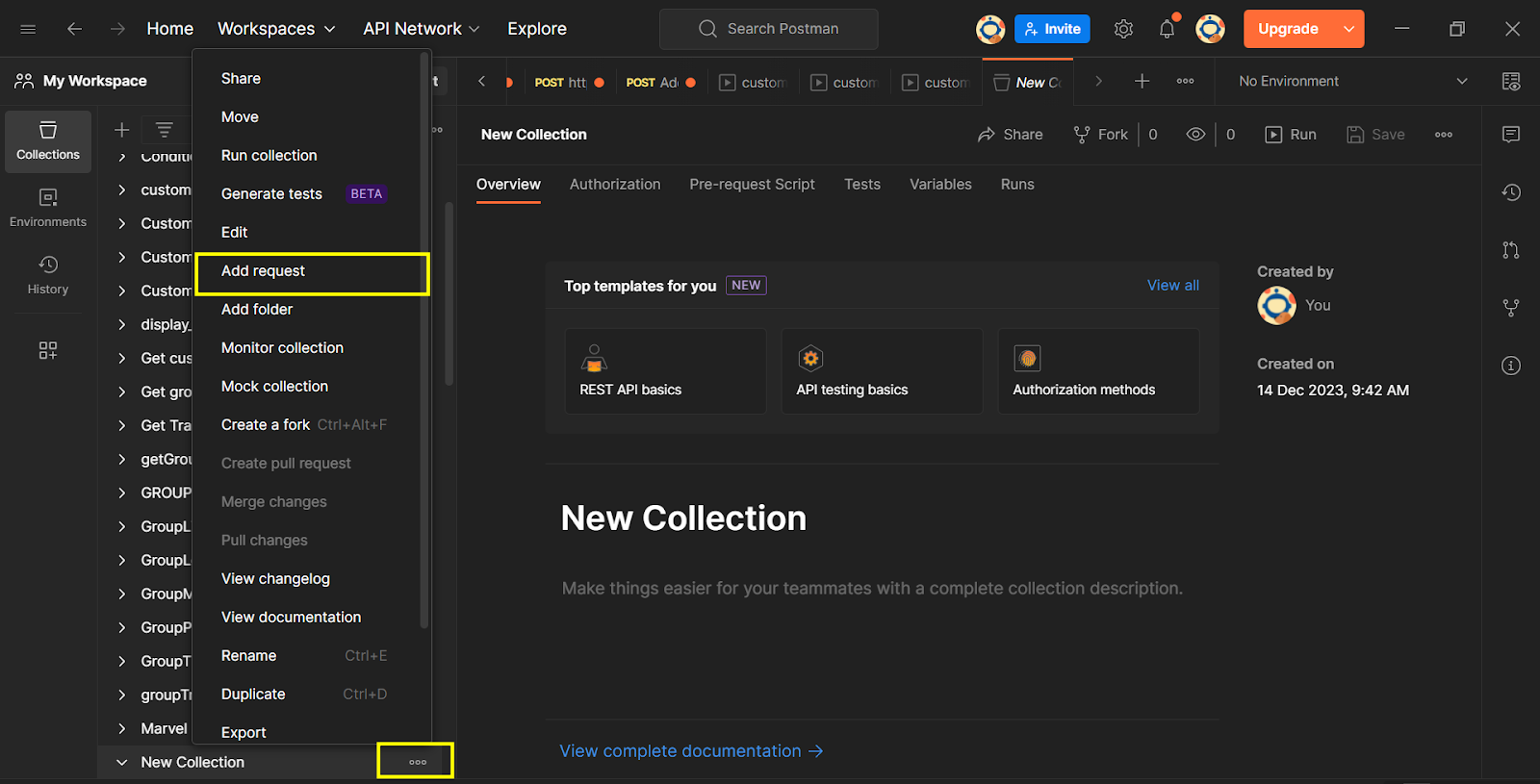
- Enter the API information such as HTTP method, API endpoint, and authorization.
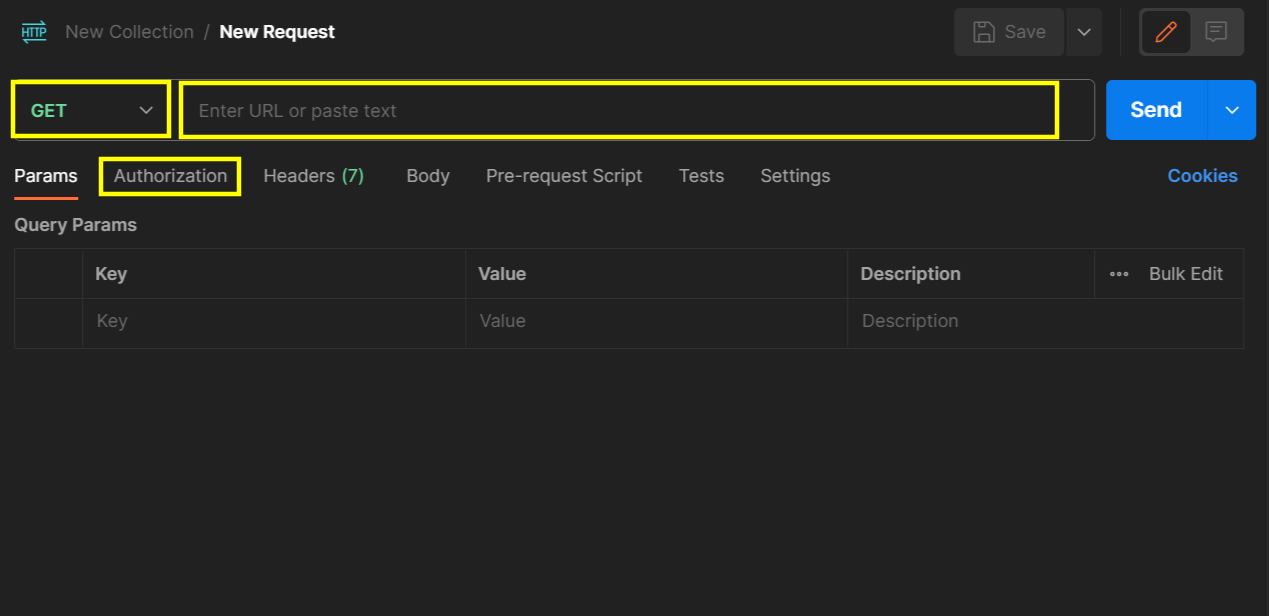
- In the Body, enter the body parameters. For the dynamic values, define the value using a variable. The variables should be defined in the format
{{variablename}}
. For example, see the below sample body of a transaction ADD API where "mobilenumber", "billNumber", and "billAmount" are the variables:
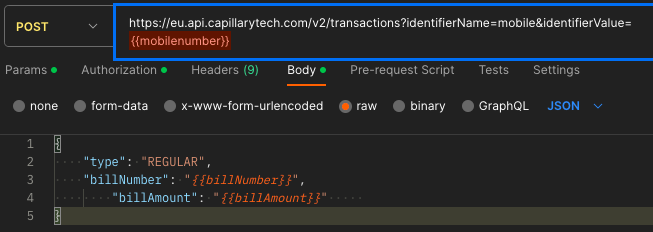
- Define the values for the variables in a CSV file and save it. Make sure that the names of the variables defined and those in the CSV files are the same. For example, if the variables are "mobilenumber", "billNumber", and "billAmount", define the values for those in the CSV file.
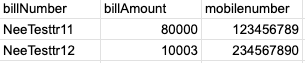
- Save the request.
- Click the three-dot menu, and click Run collection.
- Click Select File and upload the CSV file which contains the variables and the values for the dynamic parameters.
- Select the Persist response for a session checkbox to view the response in the results.
- Click Run.
- To view the responses, click on the Iterations.
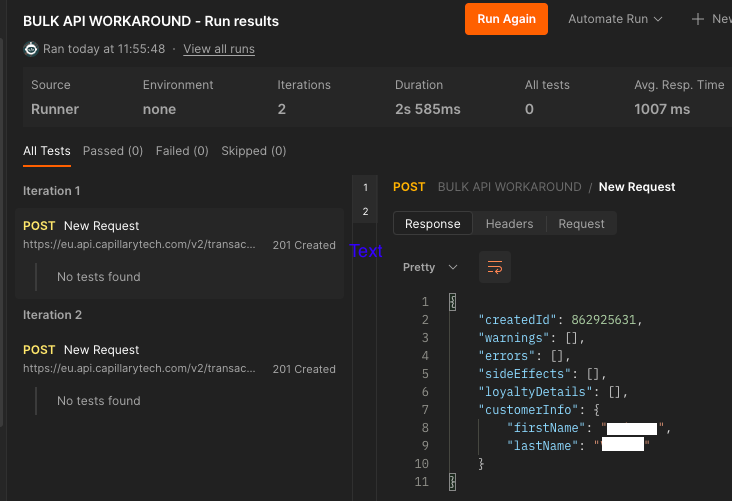
Getting started wih 'Try it' on ReadMe
This section will walk you through the steps to make your API request from the ReadMe portal.
Prerequisites
- Basic Auth credentials - till ID and MD5 encoded password. For more information refer to the Basic authentication documentation.
- Host URL
Step by step instructions
- Navigate to the Capillary developer documentation portal.
- Select the API you want to run. For example, Add a user group.
- Enter the body parameters (for POST and PUT) or query parameters (for GET and DELETE) information.
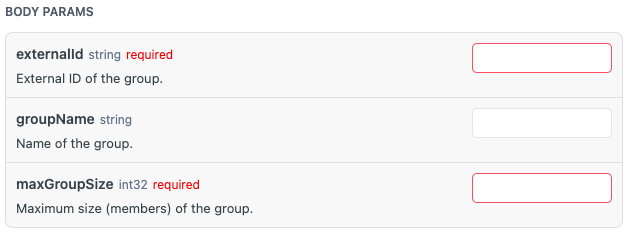
NOTE: Parameters marked required are mandatory.
For POST or PUT calls, if the body parameters fields are not available, enter the API payload in the RAW_BODY in the appropriate JSON format.

- From the CURL section, select CURL from the drop-down. To view available request CURL examples, click the REQUEST drop-down and select the CURLs.
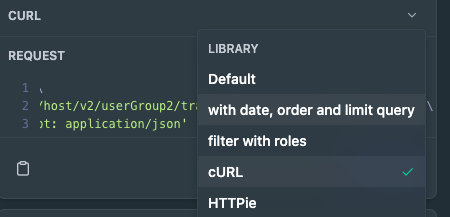
To clear an example, select Clear example.
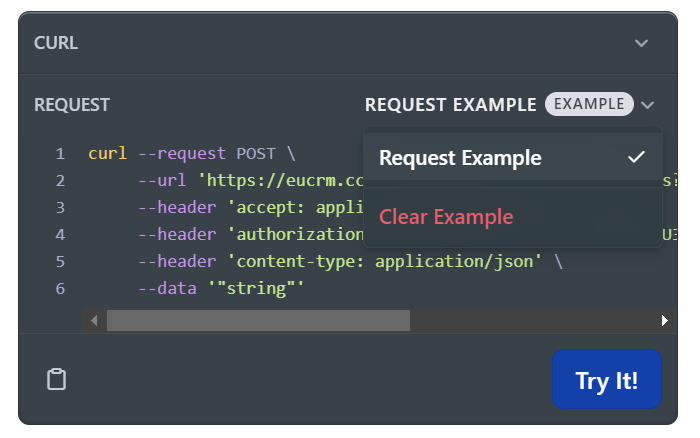
- In the URL, enter the HOST URL.
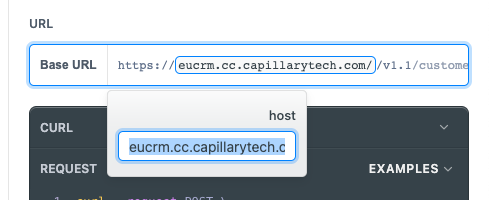
- If applicable, Enter the appropriate header values. The headers section is visible only if the API supports any additional headers.
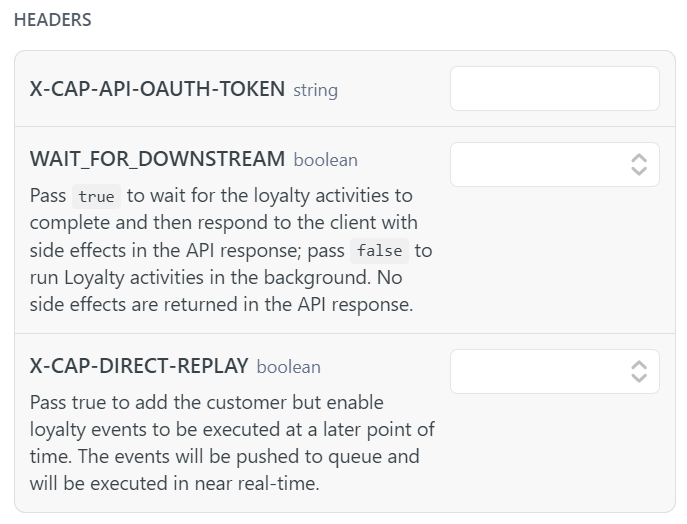
- In the Authorization section, enter the till ID and password in MD5 format.
NOTE: This is not required if you have entered the access token in theX-CAP-API-OAUTH-TOKEN
header.
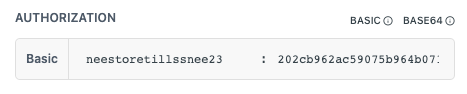
- Click Try it! . The Response section displays the response.
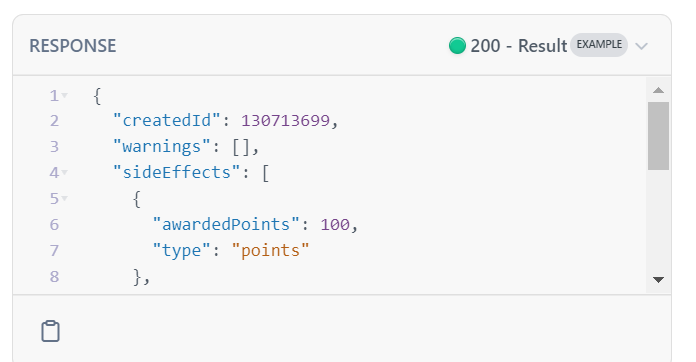
To view other sample responses, click the RESPONSE drop-down. The drop-down displays the available example responses.
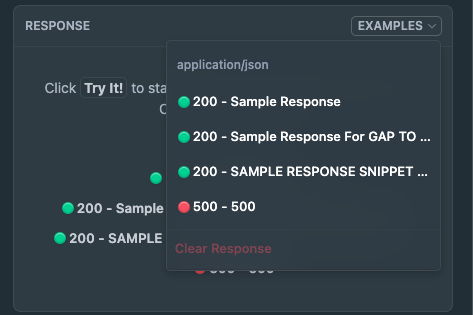
Testing API using ReadMe Try it
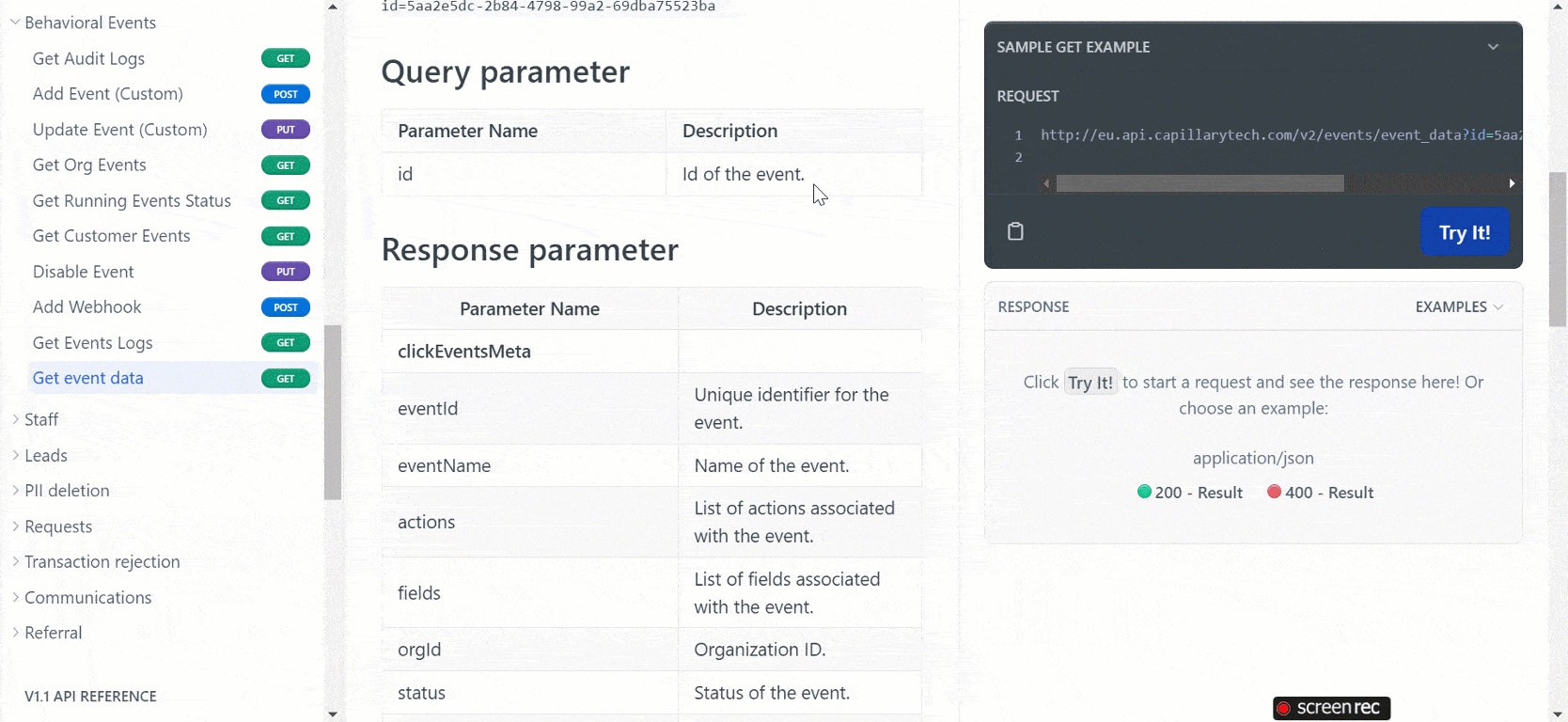
Viewing example in ReadMe
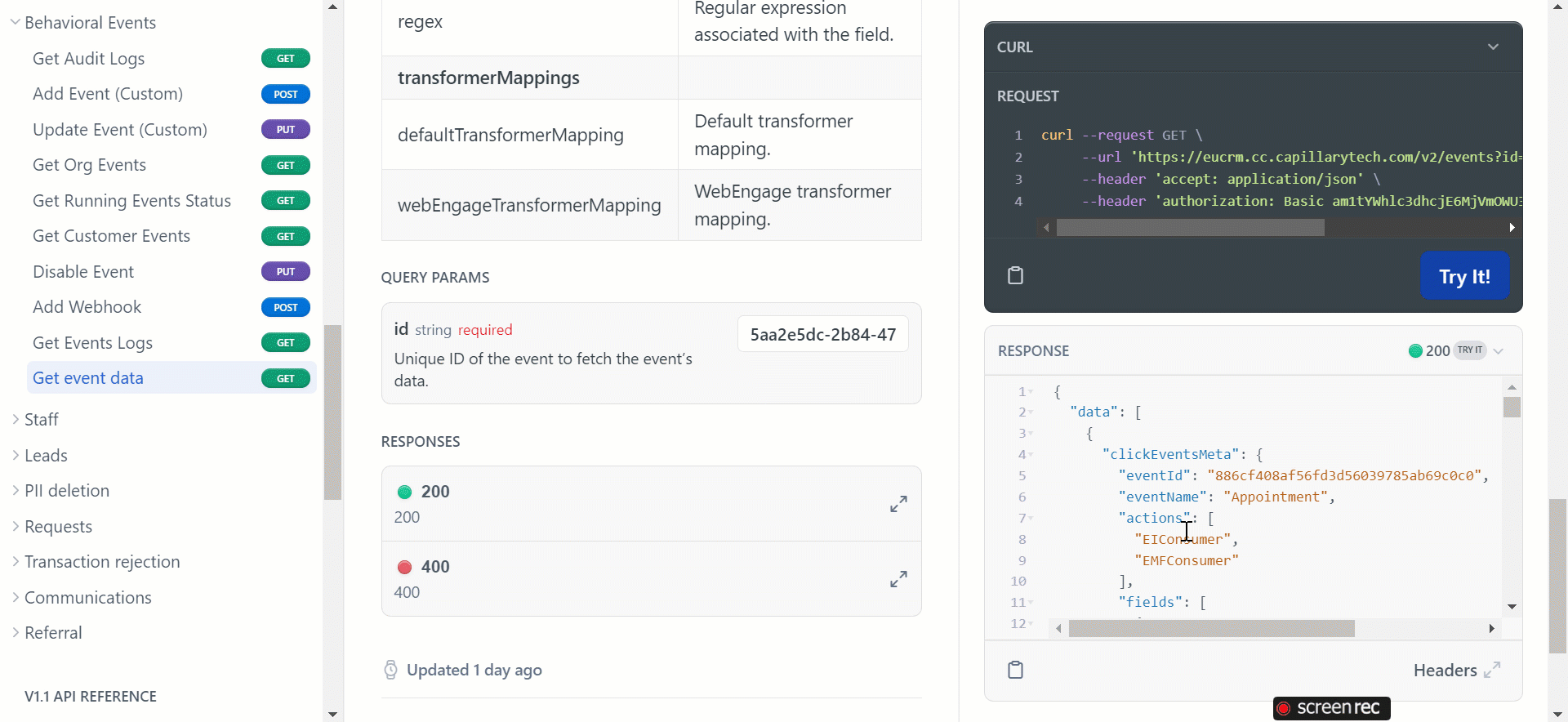
Congratulations! You have successfully sent your first Capillary API call!