Vulcan supports two categories of APIs accessible directly through the Vulcan node layer and those available through Arya APIs.
Types of APIs Supported on Vulcan
Type 1
APIs supported on the Vulcan node layer (whitelisted)
- Intouch
- XAJA
- Extension (avengers API extension.)
- Neo Extensions
- Shield / Workflow Management APIs
- Cortex APIs
Sample Method for Services/API.js:
export const methodName = (param) => {
const url = `${endpoints.vulcan_endpoint}/<intouch|neo|extensions|xaja/AjaxService>/<URL path of svc>`;
return httpRequest(url, getVulcanAPICallObject('GET'));
};
Note:
Always use
getVulcanAPICallObject
in the api.js method
Type 2
APIs supported on Arya (Node APIs and Whitelisted BE APIs):
- EMF (/loyalty/emf/v1)
- File service (/v1/file-service)
- Promo engine (/v1/promotion-management)
- Observability engine (/v1/observability)
- Rewards core (/core)
- Gamification (/gamification)
- Badges (/v1/badges)
- Any node API (loyalty/api/v1, member-care/api/v1, arya/api/v1/org-settings, arya/api/v1/creatives, any other Arya APIs)
Note:
For all the above APIs, if the endpoint has "arya/api/v1" then use
endpoints.arya_endpoint
.
Else define an endpoint in theendpoints.js
file.
Sample:
fileservice_endpoint:"https://north-america.intouch.capillarytech.com/v1/file-service"
Sample Method for Services/API.js:
export const methodName = (param) => {
const url = `${endpoints.fileservice_endpoint}/<URL path of svc>/<param>`;
return httpRequest(url, getAryaAPICallObject("GET"));
};
Note:
Always use
getAryaAPICallObject
in the api.js method.
Whitelisted Endpoints
The endpoint.js
file contains all the possible whitelisted endpoints callable from Vulcan (Type 1 and Type 2). These are based on the InTouch cluster specified during the project setup
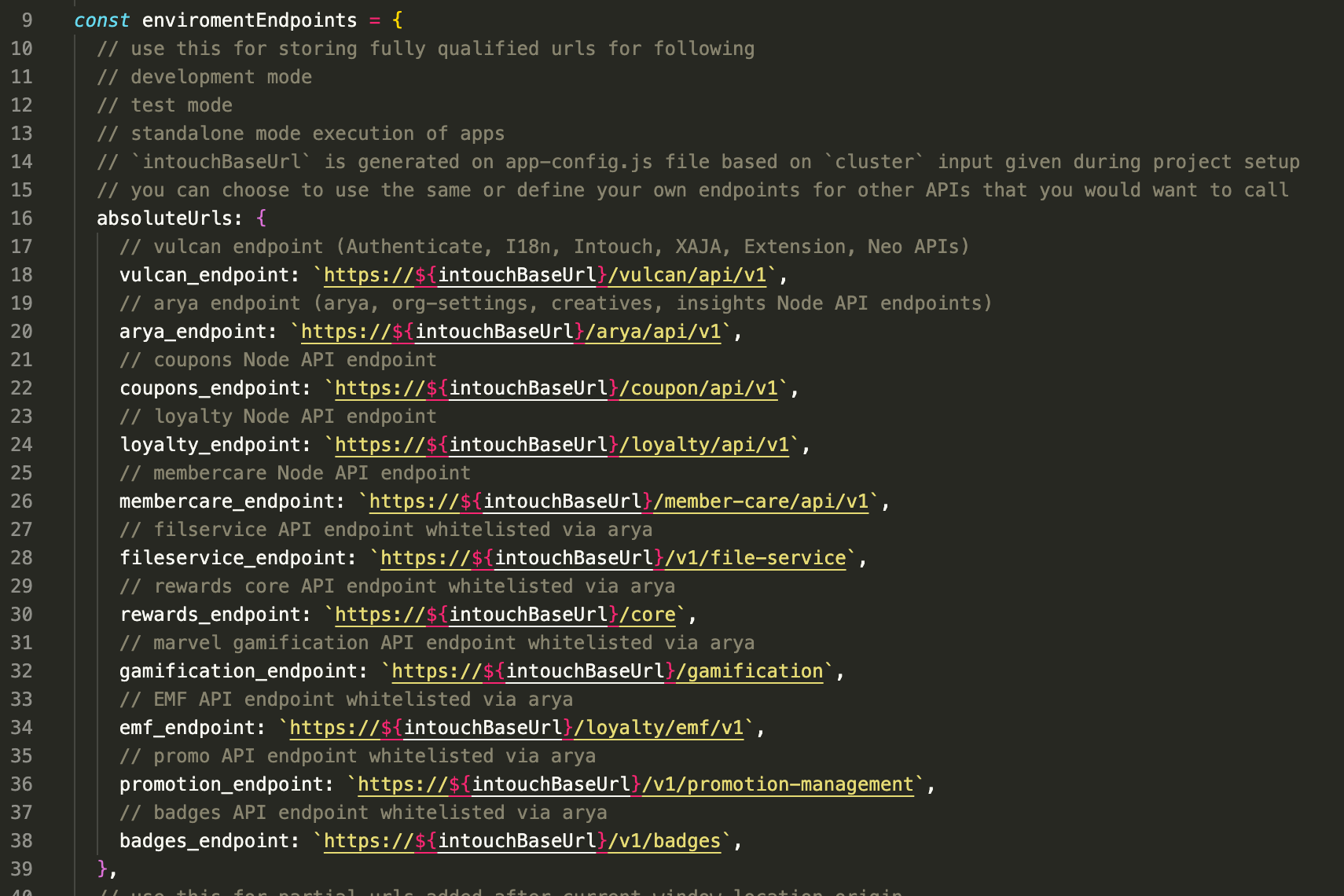
Making Vulcan endpoint calls for OAuth APIs
To make Vulcan endpoint calls for OAuth APIs, follow these steps:
- Register the OAuth client key, secret, and till ID using the
metadata/oauth-clients
API.curl --location 'https://{host}/vulcan/api/v1/metadata/oauth-clients' \ --header 'x-cap-api-auth-org-id: <orgId>' \ --header 'x-cap-remote-user: <userId>' \ --header 'Content-Type: application/json' \ --header 'Authorization: Bearer <ct_token>' \ --data '{ "key": "<client_key>", "secret": "<client_secret>", "defaultTillId": <till_id_number> }'
- After you register the OAuth client, make the necessary API calls, and ensure that the
x-cap-api-auth-type: oauth
header is included in the request.
Compressing a Vulcan API response
To improve the handling of large Vulcan API responses, enable response compression. This involves configuring the API to compress the response data and decompress the data on the client side once received. This reduces response size, improves data transfer speed, and prevents performance issues such as UI slowdowns or server-side timeouts during response parsing.
To compress an API response, follow these steps:
- Update the Vulcan SDK to version
2.3.1
. - Open the project folder on your local machine, then navigate to the
./app/services/api.js
file. - At the start of the
api.js
file, add the following code to import thedecompressJsonObject
:import { decompressJsonObject } from '@capillarytech/cap-ui-utils';
- . Add the following codes to
api.js
under thegetExtendedFields
method:- To enable response compression as
gzip
:options.headers.append('x-cap-compress-response', 'true');
- To prevent JSON parsing by the client:
options.skipParsingJson = true;
- To decompress the
gzip
response into aJSON
response:const jsonData = decompressJsonObject(data);
- To enable response compression as
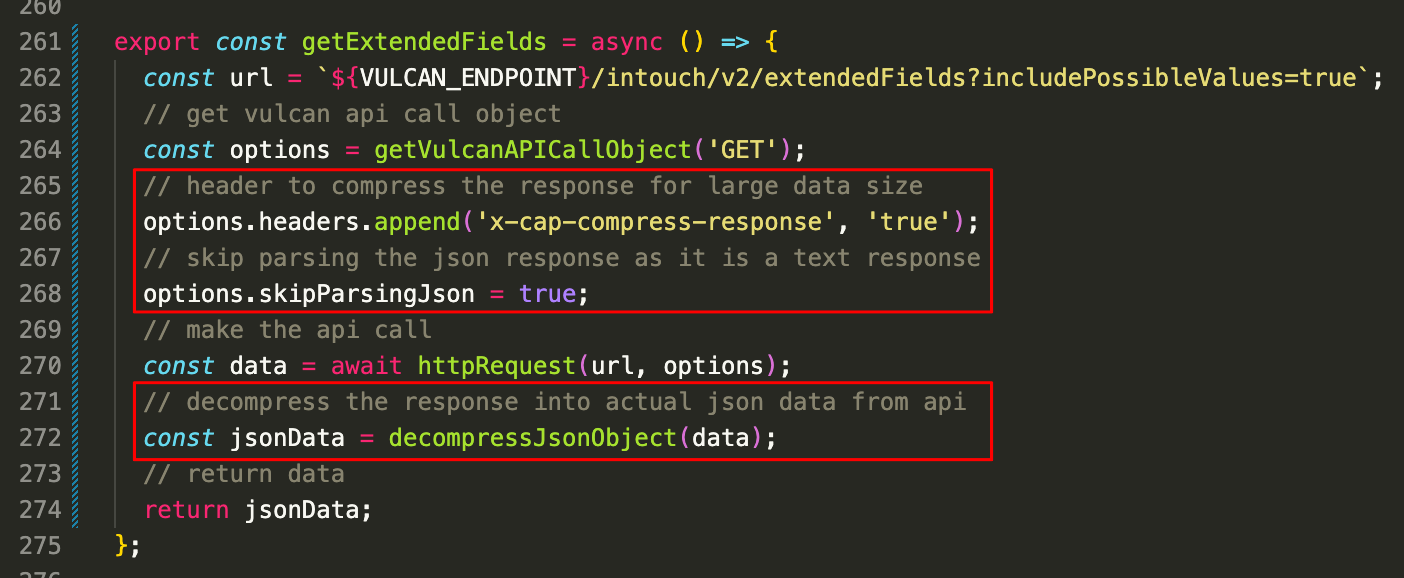
Codes to compress an API response