Understanding a Dataflow Using Aira Coder
You can ask AI to explain what a dataflow does. This helps you understand complex flows and validate logic.
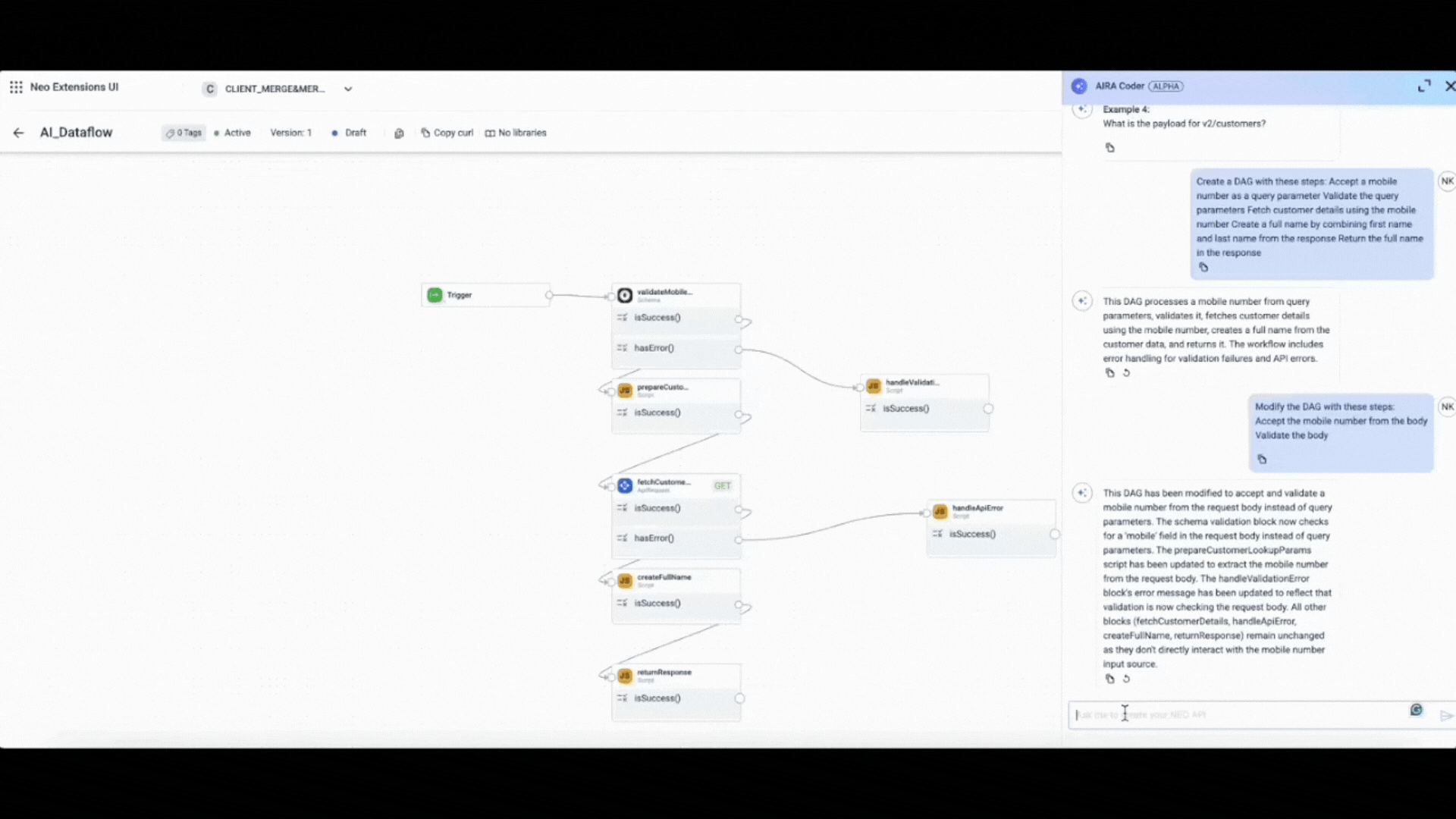
Example Prompt 1:
explain this dataflow
# Neo Dataflow Explanation: Authentication Flow
This dataflow represents a typical authentication process that exchanges client credentials for an access token. Let me explain the flow and purpose of each block:
## Overview of the Flow
The dataflow handles a client credentials authentication process with the following steps:
1. Validate client credentials from the request
2. Make an API call to obtain an authentication token
3. Process the API response and return a formatted result
4. Handle any errors that occur during the process
## Block-by-Block Explanation
### 1. `prepareAuthRequest` (Script Block)
- **Purpose**: Validates and prepares client credentials for token authentication
- **Functions**:
- Extracts `clientId` and `clientSecret` from the incoming request body
- Validates that both credentials are present
- If valid, formats them into a standardized request body for the next step
- If invalid, returns an error response
- **Outputs**:
- Success: Returns formatted credentials with proper grant type
- Error: Returns a 400 Bad Request error with details
### 2. `makeTokenApiCall` (API Request Block)
- **Purpose**: Calls an external authentication API to generate a token
- **Endpoint**: `https://nightly.api.capillarytech.com/v3/oauth/token/generate`
- **Method**: POST
- **Input**: Takes the formatted credentials from `prepareAuthRequest`
- **Headers**: Sets `Content-Type: application/json`
- **Outputs**:
- Success: Routes to `processTokenResponse`
- Error: Routes to `handleError`
### 3. `processTokenResponse` (Script Block)
- **Purpose**: Transforms the API response into a standardized client-friendly format
- **Functions**:
- Generates a unique request ID
- Extracts token information (access token, expiry time, token type)
- Formats this data into a structured response
- **Outputs**:
- Success: HTTP 200 response with token details
- Error: HTTP 401/500 response with appropriate error message
### 4. `handleError` (Script Block)
- **Purpose**: Centralized error handling for all possible failures
- **Functions**:
- Identifies which step caused the error
- Generates appropriate error messages and status codes based on error source
- Creates a standardized error response
- **Error Types Handled**:
- Preparation errors (invalid request format) - 400
- Authentication errors (invalid credentials) - 401
- General server errors - 500
## Flow Logic
1. The flow starts at `prepareAuthRequest` (marked as source)
2. If credentials are valid, it proceeds to `makeTokenApiCall`
3. If the API call succeeds, it proceeds to `processTokenResponse` to format the result
4. Any errors at any step will route to the `handleError` block for consistent error handling
This dataflow implements a robust authentication system with proper validation, error handling, and standardized response formatting.
Example Prompt 2:
explain the block prepareAuthRequest
# Block "prepareAuthRequest" in Neo
The `prepareAuthRequest` block is a Script-type block that processes authentication requests in the Neo platform. It acts as an entry point for the authentication flow and prepares the client credentials for the token generation step.
## Purpose
This block validates and transforms incoming authentication requests before sending them to an external authentication API endpoint. It extracts the client credentials from the request body and formats them in the structure expected by the authentication API.
## Key Functionality:
1. **Extracts credentials**: Pulls `clientId` and `clientSecret` from the incoming request body
2. **Validates inputs**: Checks if both required credentials are present
3. **Transforms data**: Formats the credentials into the structure expected by the authentication API
4. **Error handling**: Returns appropriate error messages if validation fails
## Data Flow:
- **Input**: Receives the initial API request with client credentials
- **Output**: When successful, outputs a formatted body with:
```json
{
"key": "[clientId value]",
"secret": "[clientSecret value]",
"grantType": "client_credentials"
}
```
## Relations:
- **onSuccess**: Flows to the `makeTokenApiCall` block which calls the external authentication API
- **onError**: Flows to the `handleError` block to format an appropriate error response
## Error Handling:
- Returns a 400 error if credentials are missing
- Returns a 500 error if any other exception occurs during execution
This block is essential for the authentication flow as it validates and prepares the request before making the actual API call to generate authentication tokens. It ensures that invalid requests are rejected early in the process with appropriate error messages.
Updated about 1 month ago