Script Block
The Script Block in a dataflow allows you to execute custom JavaScript code. You can use the Script Block to modify the dataflow to meet specific requirements, such as altering the request payload format to match an API’s expectations or applying logic to transform and enrich API responses. Access to data is managed through Data Access Object (DAO) functions and the Business logic can be defined using JavaScript methods.
Using the Script block, you can define and execute custom functions such as transforming API responses and modifying the request payload based on your requirements. It supports both single-line statements in the form of expressions and complex scripts to implement business logic.
Example Scenario
Requirement:
Consider a scenario where you need to add multiple execution paths after a validation block in a dataflow for an airline brand. The validation block validates the input against a schema in the Schema block. The result of this validation can be either success or failure, and you need to define separate execution paths for each outcome:
- If the validation succeeds: The system should retrieve customer details to proceed with issuing loyalty points.
- If the validation fails: The system should return appropriate error messages and codes.
Solution
To handle these scenarios, you can add Script blocks after the Schema block. These scripts will manage the success and failure paths.
For a Successful Validation
When the validation succeeds, use the Script block to prepare the request for fetching customer details. This involves the following steps:
- Remove Unnecessary Headers:
Certain headers can cause errors if they contain invalid values. Remove the following headers:X-cap-neo-test-variant-id
X-cap-api-attribution-entity-type
x-cap-api-attribution-entity-code
x-cap-api-attribution-till-code
- Format Query Parameters:
Format the parameters to match the expected input of the Get Customer Details API:- Set
FFN
asexternalId
to align with the API’s input format. - If the
source
parameter is missing, set it toINSTORE
.
- Set
- Send the Request:
Include the following headers and query parameters in the API request:identifierName
(e.g.,externalId
orFFN
)source
(e.g.,INSTORE
)
Below is an example of the script you can use in the Script block for the validation success scenario:
import dao from "neo/dao";
const {
getEffectiveHeaders,
getApiRequest
} = dao;
const script = {
execute: () => {
const requestQueryParams = getApiRequest().queryParams;
let requestHeaders = getEffectiveHeaders();
// Remove these headers because customer lookup api will throw error incase invalid values are passed here
delete requestHeaders["x-cap-neo-test-variant-id"];
delete requestHeaders["x-cap-api-attribution-entity-type"];
delete requestHeaders["x-cap-api-attribution-entity-code"];
delete requestHeaders["x-cap-api-attribution-till-code"];
let queryParameters = {
"identifierName" : "externalId",
"identifierValue" : requestQueryParams.FFN,
"source" : requestQueryParams.source ? requestQueryParams.source.toUpperCase() : "INSTORE"
}
return {
headers : requestHeaders,
queryParams : queryParameters
};
}
}
export {script as default}
For a Failed Validation
If the validation fails, use the Script block to generate a structured error response by,
- Retrieving validation errors
- Transforming the error into JSON format with the appropriate error code and message
- Returning an HTTP response with error details
Below is an example of the script in the Script block to handle the failure scenario.
import dao from "neo/dao";
const {
getIn,
getEffectiveHeaders
} = dao;
const script = {
execute: () => {
const validationErrors = getIn()?.err;
const errorArr = validationErrors.map((currErr) => {
return {
"status": false,
"code": 6001,
"message" : currErr.message,
"path" : currErr.instancePath
}
})
return {
http: {
"res": {
status : 400,
"json": {
"errors" : errorArr
}
}
}
}
}
}
export {script as default}
Refer to this example dataflow to understand how it is configured for a use case. Make sure that you have access to DocDemo org (100737) and access to Neo.
Configuring Script Block
To configure the Script block, perform the following:
- From the dataflow canvas, click on the connector.
- Select the Script block from the pop-up window.
- In the Block name section, enter the block name.
Note: Block names cannot contain spaces or special characters, except for underscores (_). Use camelCase or snake_case formatting. - In the Script section, write a script to meet your requirements.
- Configure the input execution logic, cachable feature, and define the execution path as per the requirement.
- Click Done.
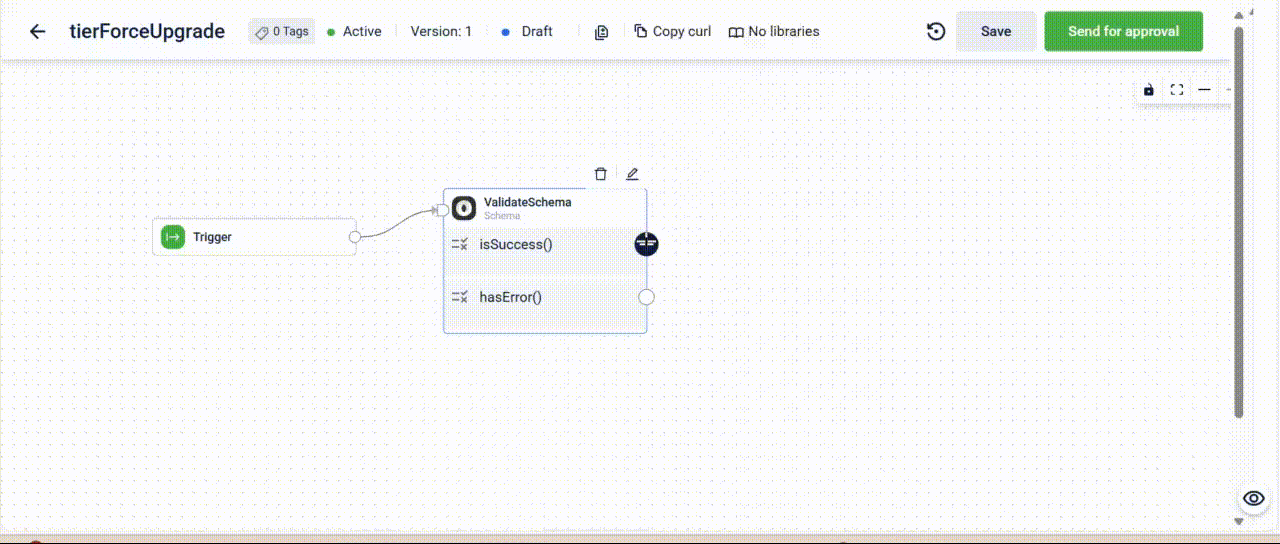
Configuring the Script block
Updated 4 days ago